一、环境搭建
1. 创建键maven项目
1. pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.gf</groupId>
<artifactId>zookeeper</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.11.1</version>
</dependency>
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.13</version>
</dependency>
</dependencies>
</project>
2. log4j.properties
log4j.rootLogger=INFO, stdout
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d %p [%c] - %m%n
log4j.appender.logfile=org.apache.log4j.FileAppender
log4j.appender.logfile.File=target/spring.log
log4j.appender.logfile.layout=org.apache.log4j.PatternLayout
log4j.appender.logfile.layout.ConversionPattern=%d %p [%c] - %m%n
二、常用API列表
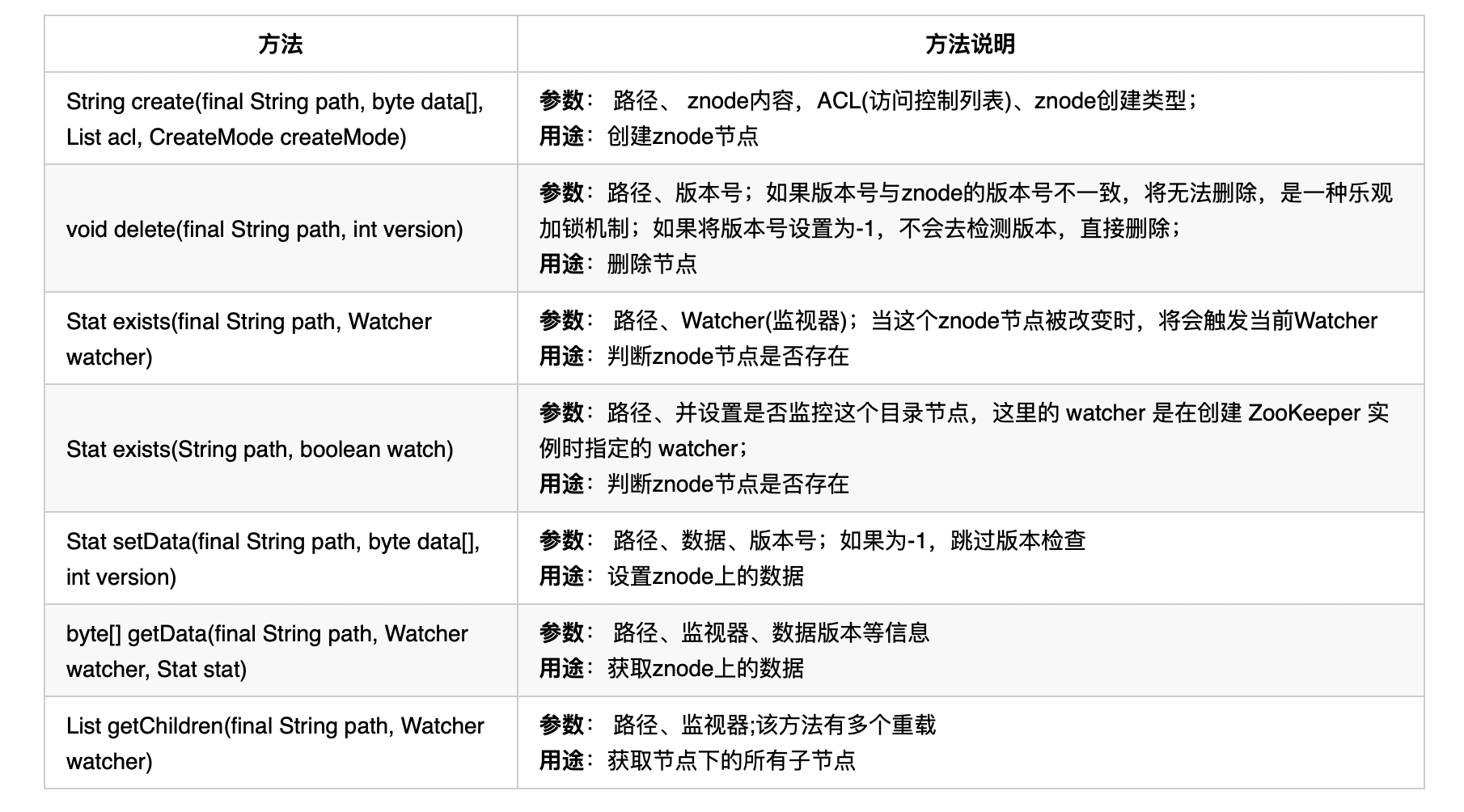
实例
package com.gf.demo1;
import java.io.IOException;
import java.util.List;
import org.apache.zookeeper.CreateMode;
import org.apache.zookeeper.KeeperException;
import org.apache.zookeeper.WatchedEvent;
import org.apache.zookeeper.Watcher;
import org.apache.zookeeper.ZooDefs.Ids;
import org.apache.zookeeper.ZooKeeper;
import org.apache.zookeeper.data.Stat;
import org.junit.Before;
import org.junit.Test;
public class TestZookeeper {
private String connectString = "127.0.0.1:2181,127.0.0.1:2182,127.0.0.1:2183";
private int sessionTimeout = 2000;
private ZooKeeper zkClient;
@Before
public void init() throws IOException {
zkClient = new ZooKeeper(connectString, sessionTimeout, new Watcher(){
public void process(WatchedEvent event) {
try {
List<String> childrens = zkClient.getChildren("/", true);
System.out.println("----------------start---------------");
for (String children : childrens) {
System.out.println(children);
}
System.out.println("-----------------end----------------");
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
//创建节点
@Test
public void createNode() throws KeeperException, InterruptedException {
String path = zkClient.create("/iiii", "hello".getBytes(), Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
System.out.println(path);
}
//获取节点下的所有子节点
@Test
public void getChildrens() throws KeeperException, InterruptedException {
List<String> childrens = zkClient.getChildren("/", false);
for (String children : childrens) {
System.out.println(children);
}
}
//删除节点
@Test
public void deleteNode() throws InterruptedException, KeeperException {
zkClient.delete("/yyyy", -1);
}
//判断节点是否存在
@Test
public void exists() throws KeeperException, InterruptedException {
Stat stat = zkClient.exists("/yyyy", false);
System.out.println(null != stat ? "exists" : "not exists");
}
//获取节点上的数据
@Test
public void getData() throws KeeperException, InterruptedException {
byte[] data = zkClient.getData("/yyyy", false, null);
System.out.println(new String(data));
}
}
评论